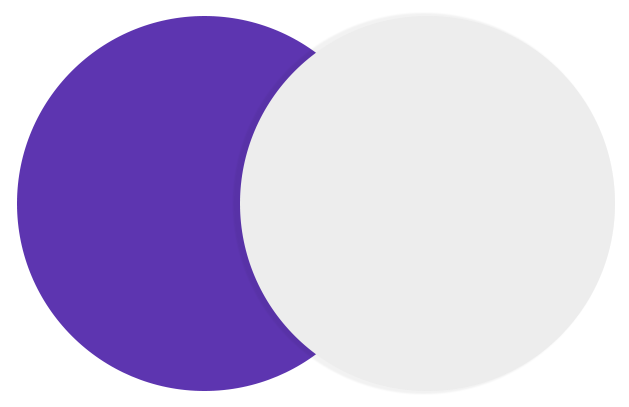
값이나 객체를 JSON 문자열로 변환하는 JSON.stringify()
JSON.stringify() 메서드는 JavaScript 값이나 객체를 JSON 문자열로 변환하는 ES6 문법입니다. 선택적으로, replacer를 함수로 전달할 경우 변환 전 값을 변형할 수 있고, 배열로 전달할 경우 지정한 속성만 결과에 포함합니다.
- 배열이 아닌 객체의 속성들은 어떤 특정한 순서에 따라 문자열화 될 것이라고 보장되지 않는다. 같은 객체의 문자열화에 있어서 속성의 순서에 의존하지 않는다.
- 열거 불가능한 속성들은 무시된다
console.log(JSON.stringify({ x: 5, y: 6 }));
// expected output: "{"x":5,"y":6}"
// undefined, 함수, 심볼(symbol)은 변환될 때 생략되거나(객체 안에 있을 경우) null 로 변환된다(배열 안에 있을 경우).
console.log(JSON.stringify({ x: [10, undefined, function(){}, Symbol('')] }));
// expected output: "{"x":[10,null,null,null]}"
console.log(JSON.stringify(new Date(2006, 0, 2, 15, 4, 5)));
// expected output: ""2006-01-02T15:04:05.000Z""
※ replacer 매개 변수
replacer 매개변수는 함수 또는 배열이 될 수 있다. 함수일 때는 문자열화 될 key 와 value, 2개의 매개변수를 받는다.
// 함수에 대한 예제
function replacer(key, value) {
if (typeof value === "string") {
// undefined 를 반환하면, 그 속성은 JSON 문자열 결과에 포함되지 않는다.
return undefined;
}
return value;
}
var foo = {foundation: "Mozilla", model: "box", week: 45, transport: "car", month: 7};
var jsonString = JSON.stringify(foo, replacer);
// JSON 문자열 결과는 {"week":45,"month":7} 이다.
// 배열에 대한 예제
// replacer 가 배열인 경우, 그 배열의 값은 JSON 문자열의 결과에 포함되는 속성의 이름을 나타낸다.
JSON.stringify(foo, ['week', 'month']);
// '{"week":45,"month":7}', 단지 "week" 와 "month" 속성을 포함한다
※ space 매개 변수
space 매개변수는 최종 문자열의 간격을 제어한다. 숫자일 경우 최대 10자 만큼의 공백 문자 크기로 들여쓰기되며, 문자열인 경우 해당 문자열 또는 처음 10자 만큼 들여쓰기 된다.
JSON.stringify({ a: 2 }, null, ' ');
// '{
// "a": 2
// }'
// '\t'를 사용하면 일반적으로 들여쓰기 된 코드스타일과 유사함.
JSON.stringify({ uno: 1, dos: 2 }, null, '\t');
// returns the string:
// '{
// "uno": 1,
// "dos": 2
// }'
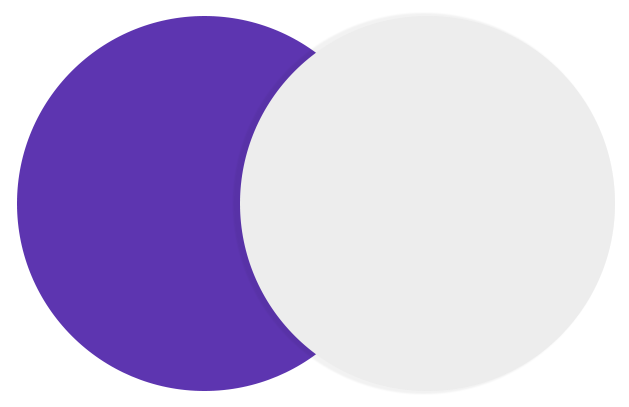
JSON 문자열을 분석해 값이나 객체를 생성하는 JSON.parse()
JSON.parse() 메서드는 JSON 문자열의 구문을 분석하고, 그 결과에서 JavaScript 값이나 객체를 생성합니다. 선택적으로, reviver 함수를 인수로 전달할 경우, 결과를 반환하기 전에 변형할 수 있습니다.
const json = '{"result":true, "count":42}';
const obj = JSON.parse(json);
console.log(obj.count);
// expected output: 42
console.log(obj.result);
// expected output: true
※ reviver 매개 변수
reviver가 주어지면 분석한 값을 반환하기 전에 변환합니다. 구체적으로는, 분석한 값과 그 모든 속성(가장 깊게 중첩된 속성부터 시작해 제일 바깥의 원래 값까지)을 각각 reviver에 전달합니다.
JSON.parse('{"p": 5}', (key, value) =>
typeof value === 'number'
? value * 2 // 숫자라면 2배
: value // 나머진 그대로
);
// { p: 10 }
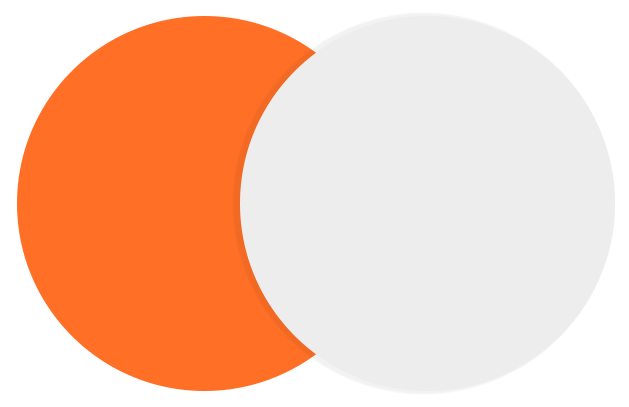
localStorage 사용 예
// Creating an example of JSON
var session = {
'screens': [],
'state': true
};
session.screens.push({ 'name': 'screenA', 'width': 450, 'height': 250 });
session.screens.push({ 'name': 'screenB', 'width': 650, 'height': 350 });
session.screens.push({ 'name': 'screenC', 'width': 750, 'height': 120 });
session.screens.push({ 'name': 'screenD', 'width': 250, 'height': 60 });
session.screens.push({ 'name': 'screenE', 'width': 390, 'height': 120 });
session.screens.push({ 'name': 'screenF', 'width': 1240, 'height': 650 });
// Converting the JSON string with JSON.stringify()
// then saving with localStorage in the name of session
localStorage.setItem('session', JSON.stringify(session));
// Example of how to transform the String generated through
// JSON.stringify() and saved in localStorage in JSON object again
var restoredSession = JSON.parse(localStorage.getItem('session'));
// Now restoredSession variable contains the object that was saved
// in localStorage
console.log(restoredSession);

출처
[JSON.stringify()] - https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/JSON/stringify
[JSON.parse()] - https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Statements/continue
'① 기본 > javascript' 카테고리의 다른 글
[JS] for/while/switch문 break와 continue 차이 그리고 label (0) | 2021.01.26 |
---|---|
ES11 자주 사용하는 문법 정리 (0) | 2020.11.18 |
ES6 자주 사용하는 문법 정리 (0) | 2020.10.12 |
ES5 자주 사용하는 문법 정리 (1) | 2020.10.07 |