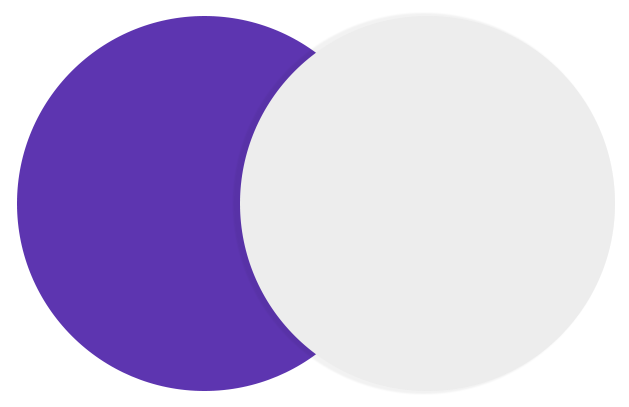
Array.isArray()
Array.isArray() 메서드는 인자가 Array인지 판별합니다.
Array.isArray([1, 2, 3]); // true
Array.isArray(Array.prototype); // true Array.prototype은 스스로도 배열입니다
Array.isArray({foo: 123}); // false
Array.isArray('foobar'); // false
Array.isArray(undefined); // false
// instanceof vs isArray
// Array 객체를 판별할 때, Array.isArray는 iframe을 통해서도 작동하기 때문에 instanceof 보다 적합합니다.
// 아래 코드를 실행하면 지원하지 않는 환경에서도 Array.isArray()를 사용할 수 있습니다.
if (!Array.isArray) {
Array.isArray = function(arg) {
return Object.prototype.toString.call(arg) === '[object Array]';
};
}
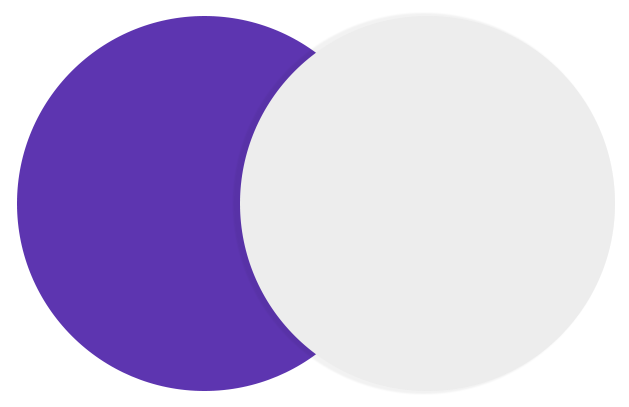
Array.prototype.indexOf()
indexOf() 메서드는 배열에서 지정된 요소를 찾을 수 있는 첫 번째 인덱스를 반환하고 존재하지 않으면 -1을 반환합니다. indexOf()는 엄격한 동등성 (=== 또는 triple-equals 연산자에서 사용하는 것과 같은 메서드)을 사용하여 검색 요소를 Array의 요소와 비교합니다. 제공된 색인 값이 음수이면 배열 끝에서부터의 오프셋 값으로 사용됩니다. 참고 : 제공된 색인이 음수이면 배열은 여전히 앞에서 뒤로 검색됩니다. 계산 된 인덱스가 0보다 작 으면 전체 배열이 검색됩니다. 기본값 : 0 (전체 배열 검색).
// 구문 arr.indexOf(searchElement[, fromIndex])
const beasts = ['ant', 'bison', 'camel', 'duck', 'bison'];
console.log(beasts.indexOf('bison'));
// expected output: 1
// start from index 2 , 검색을 시작할 색인
console.log(beasts.indexOf('bison', 2));
// expected output: 4
console.log(beasts.indexOf('giraffe'));
// expected output: -1
// 요소의 모든 항목 찾기
var indices = [];
var array = ['a', 'b', 'a', 'c', 'a', 'd'];
var element = 'a';
var idx = array.indexOf(element);
while (idx != -1) {
indices.push(idx);
idx = array.indexOf(element, idx + 1);
}
console.log(indices);
// [0, 2, 4]
//요소가 배열에 존재하는지 확인하고 배열을 업데이트
function updateVegetablesCollection (veggies, veggie) {
if (veggies.indexOf(veggie) === -1) {
veggies.push(veggie);
console.log('새로운 veggies 컬렉션 : ' + veggies);
} else if (veggies.indexOf(veggie) > -1) {
console.log(veggie + ' 은 이미 veggies 컬렉션에 존재합니다.');
}
}
var veggies = ['potato', 'tomato', 'chillies', 'green-pepper'];
updateVegetablesCollection(veggies, 'spinach');
// 새로운 veggies 컬렉션 : potato, tomato, chillies, green-pepper, spinach
updateVegetablesCollection(veggies, 'spinach');
// spinach 은 이미 veggies 컬렉션에 존재합니다.
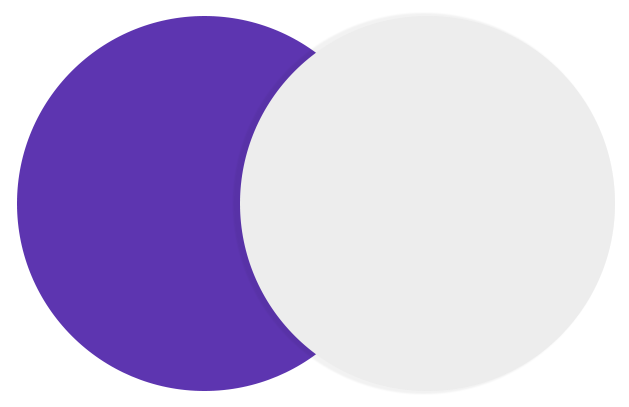
String.prototype.indexOf()
indexOf() 메서드는 호출한 String 객체에서 주어진 값과 일치하는 첫 번째 인덱스를 반환합니다. 일치하는 값이 없으면 -1을 반환합니다. 문자열에서 찾기 시작하는 위치를 나타내는 인덱스 값입니다. 어떤 정수값이라도 가능합니다. 기본값은 0이며, 문자열 전체를 대상으로 찾게 됩니다. 만약 fromIndex 값이 음의 정수이면 전체 문자열을 찾게 됩니다. 만약 fromIndex >= str.length 이면, 검색하지 않고 바로 -1을 반환합니다.
// 구문 str.indexOf(searchValue[, fromIndex])
const paragraph = 'The quick brown fox jumps over the lazy dog. If the dog barked, was it really lazy?';
const searchTerm = 'dog';
const indexOfFirst = paragraph.indexOf(searchTerm);
console.log(`The index of the first "${searchTerm}" from the beginning is ${indexOfFirst}`);
// expected output: "The index of the first "dog" from the beginning is 40"
console.log(`The index of the 2nd "${searchTerm}" is ${paragraph.indexOf(searchTerm, (indexOfFirst + 1))}`);
// expected output: "The index of the 2nd "dog" is 52"
// indexOf() 메서드는 대소문자를 구분합니다. 예를 들면, 아래 예제는 일치하는 문자열이 없으므로 -1을 반환합니다.
'Blue Whale'.indexOf('blue'); // returns -1
// indexOf()를 사용하여 문자열 내의 특정 문자 숫자 세기
var str = 'To be, or not to be, that is the question.';
var count = 0;
var pos = str.indexOf('e'); //pos는 4의 값을 가집니다.
while (pos !== -1) {
count++;
pos = str.indexOf('e', pos + 1); // 첫 번째 e 이후의 인덱스부터 e를 찾습니다.
}
console.log(count); // 로그에 4를 출력합니다.
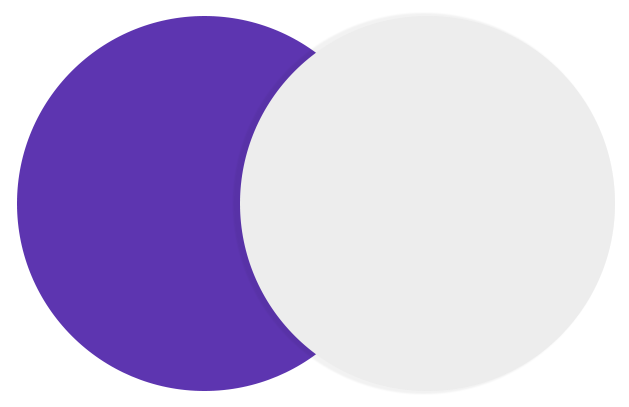
Array.protorype.map()
map() 메서드는 배열 내의 모든 요소 각각에 대하여 주어진 함수를 호출한 결과를 모아 새로운 배열을 반환합니다.
// 구문 arr.map((currentValue, index, array)=> {});
const array1 = [1, 4, 9, 16];
// pass a function to map
const map1 = array1.map(x => x * 2);
console.log(map1);
// expected output: Array [2, 8, 18, 32]
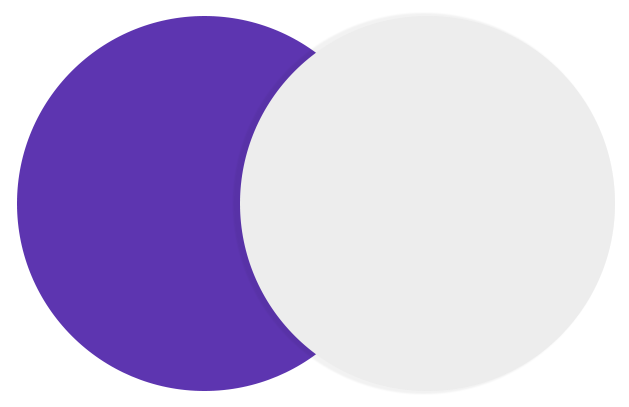
Array.prototype.filter()
filter() 메서드는 주어진 함수의 테스트를 통과하는 모든 요소를 모아 새로운 배열로 반환합니다.
const words = ['spray', 'limit', 'elite', 'exuberant', 'destruction', 'present'];
const result = words.filter(word => word.length > 6);
console.log(result);
// expected output: Array ["exuberant", "destruction", "present"]
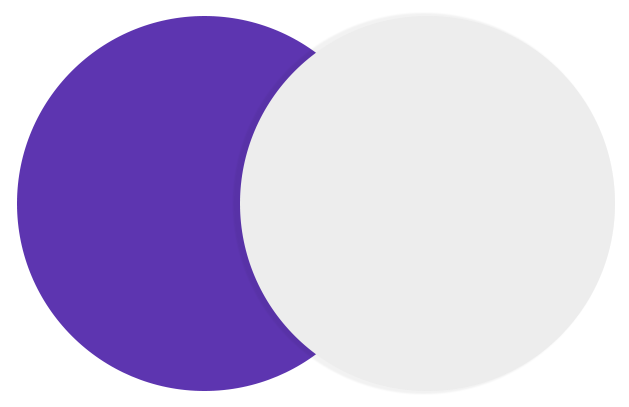
Array.prototype.reduce();
reduce() 메서드는 배열의 각 요소에 대해 주어진 리듀서(reducer) 함수를 실행하고, 하나의 결과값을 반환합니다.
const array1 = [1, 2, 3, 4];
const reducer = (accumulator, currentValue) => accumulator + currentValue;
// 1 + 2 + 3 + 4
console.log(array1.reduce(reducer));
// expected output: 10
// 5 + 1 + 2 + 3 + 4
console.log(array1.reduce(reducer, 5));
// expected output: 15
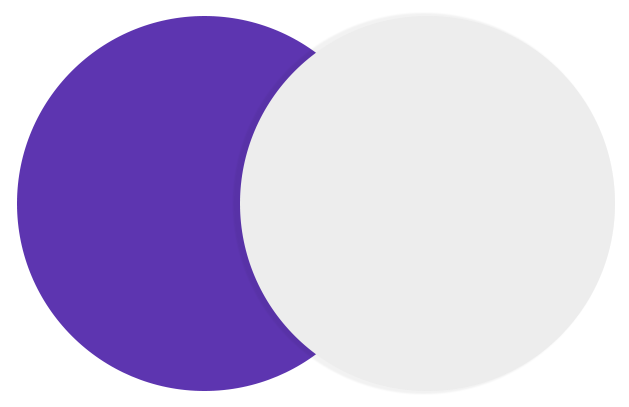
Array.prototype.forEach();
forEach() 메서드는 주어진 함수를 배열 요소 각각에 대해 실행합니다.
const array1 = ['a', 'b', 'c'];
array1.forEach(element => console.log(element));
// expected output: "a"
// expected output: "b"
// expected output: "c"
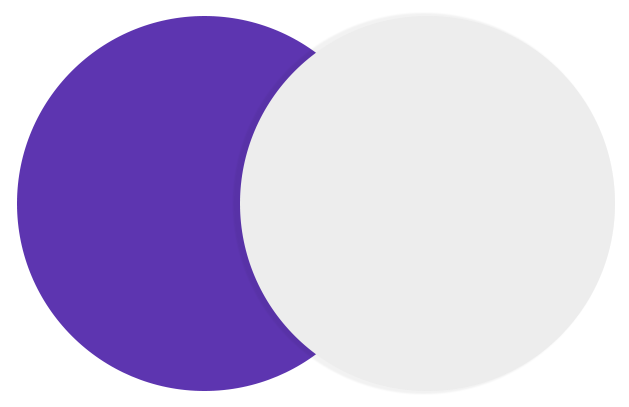
Array.prototype.some()
some() 메서드는 배열 안의 어떤 요소라도 주어진 판별 함수를 통과하는지 테스트합니다.
const array = [1, 2, 3, 4, 5];
// checks whether an element is even
const even = (element) => element % 2 === 0;
console.log(array.some(even));
// expected output: true
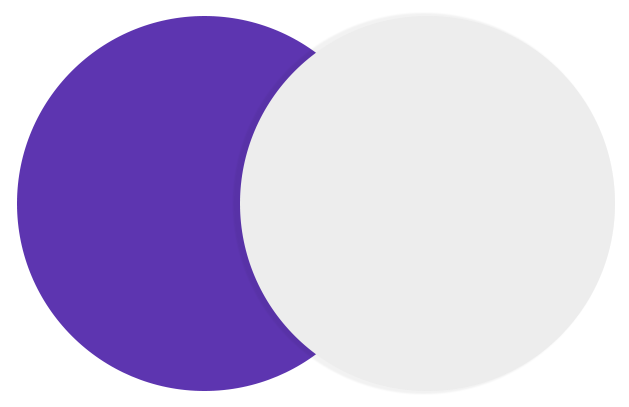
Array.prototype.every();
every() 메서드는 배열 안의 모든 요소가 주어진 판별 함수를 통과하는지 테스트합니다.
// 참고: 빈 배열에서 호출하면 무조건 true를 반환합니다.
const isBelowThreshold = (currentValue) => currentValue < 40;
const array1 = [1, 30, 39, 29, 10, 13];
console.log(array1.every(isBelowThreshold));
// expected output: true
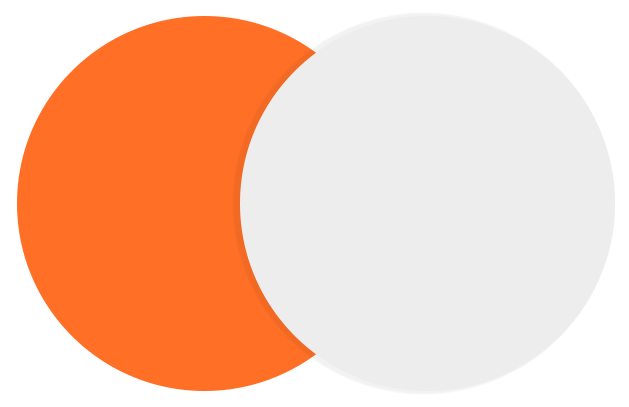
결론
자주 사용하는 ES5 문법이 더 있다면 댓글로 남겨주세요~!

출처
[Array.isArray] - developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Array/isArray
[Array.protorype.indexOf()] - developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Array/indexOf
[String.protorype.indexOf()] - developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/String/indexOf
[Array.protorype.map()] - developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Array/map
[Array.protorype.filter()] - developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Array/filter
[Array.protorype.reduce()] - https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Array/Reduce
[Array.protorype.forEach()] - https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Array/forEach
[Array.protorype.some()] - https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Array/some
[Array.protorype.every()] - https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Array/every
'① 기본 > javascript' 카테고리의 다른 글
[JS] JSON.stringify() 와 JSON.parse() (2) | 2021.01.30 |
---|---|
[JS] for/while/switch문 break와 continue 차이 그리고 label (0) | 2021.01.26 |
ES11 자주 사용하는 문법 정리 (0) | 2020.11.18 |
ES6 자주 사용하는 문법 정리 (0) | 2020.10.12 |